djangoでhtml形式のメールを送信してみる
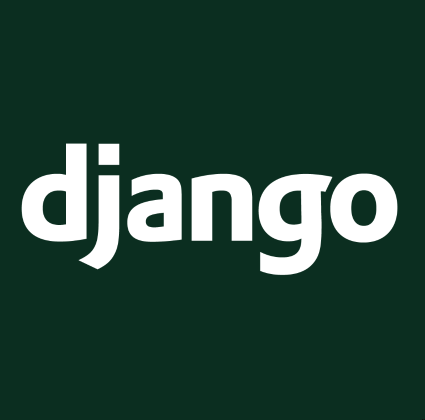
djangoでhtml形式のメールを送信してみる
参考:
・DjangoでHTMLメールを送信する
・djangoでメールを送信する
階層
.
└── django_project
├── config
│ ├── settings.py
│ └── urls.py
├── manage.py
├── myapp
│ ├── urls.py
│ └── views.py
└── templates
└── mail
├── sample.html
└── sample.txt
プログラム
【メールの設定】
settings.pyに下記を追記します。
詳細は「参考」に記載してあります。
# config/settings.py
# コンソールに表示する場合は下記の通り
EMAIL_BACKEND = 'django.core.mail.backends.console.EmailBackend'
# 実際にメールを送信する場合は下記の通り
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
# 送信元のアドレスを指定
DEFAULT_FROM_EMAIL = 'your_mail_address@gmail.com'
# gmailの場合固定
EMAIL_HOST = 'smtp.gmail.com'
# gmailの場合固定
EMAIL_PORT = 587
# あなたのgmailメールアドレス
EMAIL_HOST_USER = 'your_mail_address@gmail.com'
# あなたのgmailのパスワード又はアプリ用パスワード
EMAIL_HOST_PASSWORD = 'xxxxxxxxxxxxxxxx'
# 暗号化するのでTrue
EMAIL_USE_TLS = True
【ルーティング】
# config/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('myapp/', include('myapp.urls')),
]
# myapp/urls.py
from django.urls import path
from . import views
app_name = 'myapp'
urlpatterns = [
path('notify/', views.notify, name='notify'),
]
【ビュー】
render_to_string() - テンプレートファイルを取得しています。
context - html内に埋め込みたい値をに格納しています。
html_message - メールの内容にHTMLを指定しています。
from django.template.loader import render_to_string
from django.core.mail import send_mail
from django.shortcuts import render
def notify(request):
context = {
'name': 'John',
'age':'20',
'comment':'おめでとう',
}
subject = "subject"
message = render_to_string('mail/sample.txt', context)
from_email = "your_mail_address@gmail.com"
recipient_list = [
"recipient_mail_address@gmail.com"
]
html_message = render_to_string('mail/sample.html', context)
send_mail(subject, message, from_email, recipient_list, html_message=html_message)
return render(request, 'myapp/index.html')
【HTML】
<!--templates/mail/sample.html-->
<html>
<body>
<div style="color: #FF0000;">こんにちは{{ name }}さん。</div>
<div style="color: #0000FF;">{{ age }}の誕生日、</div>
<div style="color: #008000;">{{ comment }}。</div>
</body>
</html>
結果
きちんとHTMLが利いているっぽいですね。
YouTube
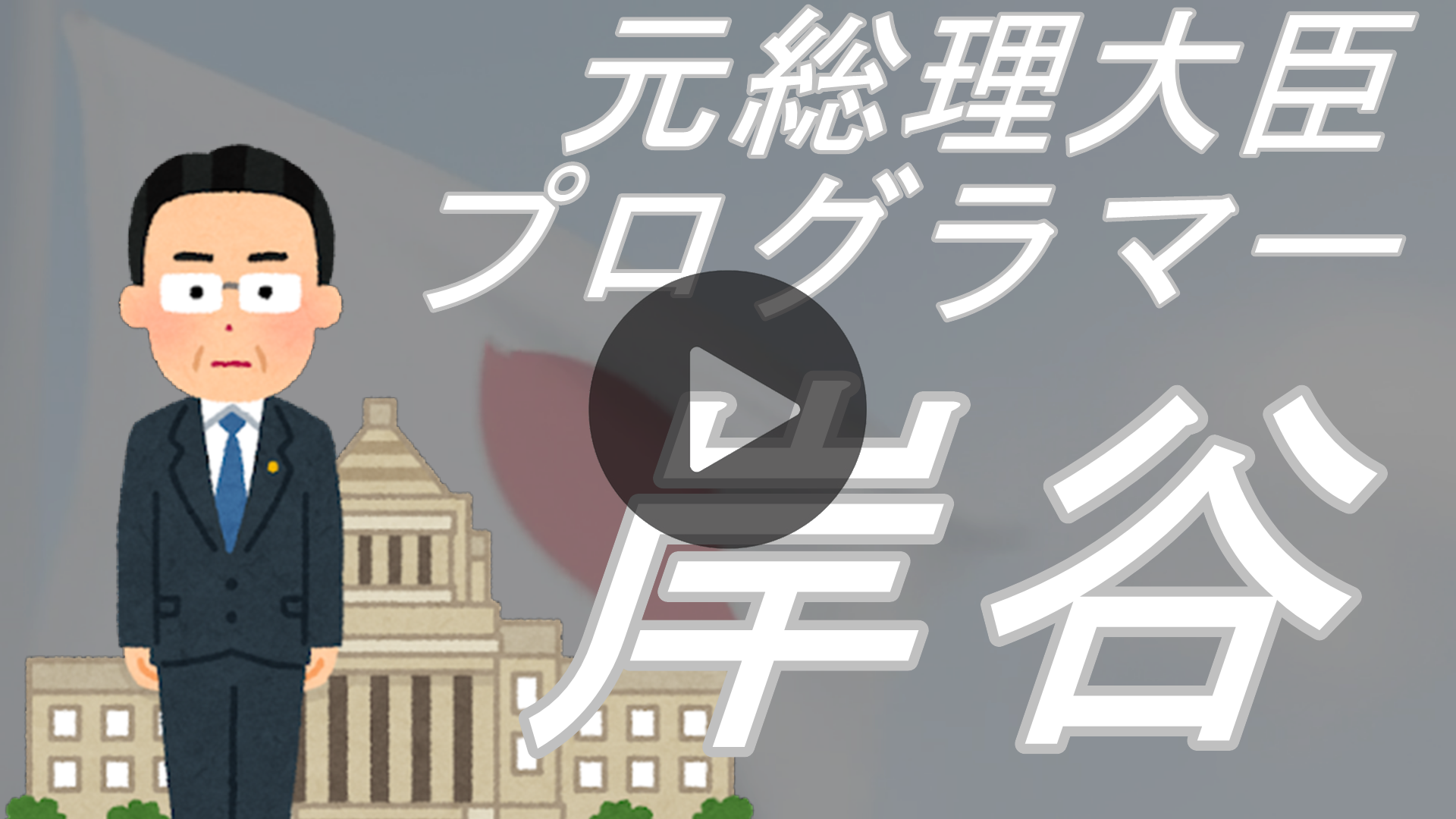
ディスカッション
コメント一覧
まだ、コメントがありません