function と アロー関数 と this と that について
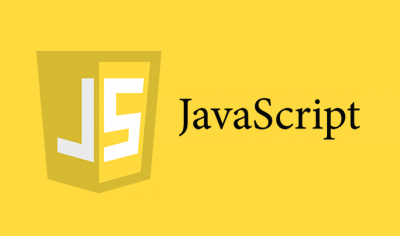
javascript の function アロー関数 this that の挙動を一度まとめておきます。
パターン1 function 内に function
function 内の this は自分自身を指します。
function 内の function も同様に自分自身を指します。
value = 'window'
let myObject = {
value: 'my',
myFunc: function() {
console.log(this); // {value: "my", myFunc: ƒ}
console.log(this.value); // my
//---------------------
let ourObject = {
value: 'our',
ourFunc: function(){
console.log(this); // {value: "our", ourFunc: ƒ}
console.log(this.value); // our
}
}
ourObject.ourFunc();
//---------------------
}
};
myObject.myFunc();
パターン2 アロー関数 内に アロー関数
アロー関数内の this は呼び出し元 window を指します。
例えアロー関数内のアロー関数でもそれは一緒です。
value = 'window'
let yourObject = {
value: 'your',
yourFunc: () => {
console.log(this); // Window {window: Window, self: Window, document: document, name: "", location: Location, …}
console.log(this.value); // window
//---------------------
let theirObject = {
value: 'their',
theirFunc: () => {
console.log(this); // Window {window: Window, self: Window, document: document, name: "", location: Location, …}
console.log(this.value); // window
}
}
theirObject.theirFunc();
//---------------------
}
};
yourObject.yourFunc();
パターン3 function 内に アロー関数
helloFunc は function 内なので this は自分自身を指します。
これはパターン 1 と一緒です。
worldFunc の呼び出し元は function なので helloFunc になります。
これはパターン2 と違う所です。
value = 'window'
let helloObject = {
value: 'hello',
helloFunc: function () {
console.log(this) // {value: "hello", helloFunc: ƒ}
console.log(this.value); // hello
//---------------------
let worldObject = {
value: 'world',
worldFunc: () => {
console.log(this); // {value: "hello", helloFunc: ƒ} ※ function 内のアロー関数内なので this は helloObject まで戻る
console.log(this.value); // hello
}
}
worldObject.worldFunc();
//---------------------
}
}
helloObject.helloFunc();
that を使ってみる
下記のコードはパターン1 の function 内の function です。
goodbyeObject 内で「say」を出力したい場合があるとしますが、「this.value」を goodbyeFunc 内で使用すると「goodbye」が出力されてしまいます。
「this.value が say の状態」の時に、this を変数 that に入れ that を goodbyeObject 内で使用する事で実現出来ます。
value = 'window'
let sayObject = {
value: 'say',
sayFunc: function() {
that = this
console.log(this); // {value: "say", sayFunc: ƒ}
console.log(this.value); // say
//---------------------
let goodbyeObject = {
value: 'goodbye',
goodbyeFunc: function(){
console.log(this); // {value: "goodbye", goodbyeFunc: ƒ}
console.log(this.value); // goodbye
console.log(that) // {value: "say", sayFunc: ƒ}
console.log(that.value) // say
}
}
goodbyeObject.goodbyeFunc();
//---------------------
}
};
sayObject.sayFunc();
ディスカッション
コメント一覧
まだ、コメントがありません