djangoの自動テストでrequestを送ってみる(Client)
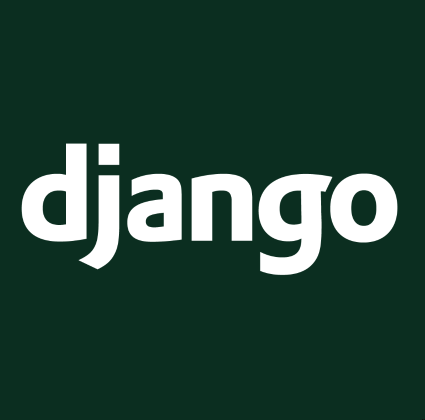
djangoの自動テストでrequestを送ってみる(Client)
djangoでテストをする時にrequestを送る方法を書き残しておきたいと思います。Clientオブジェクトを使う事で実現できます。
参考:
・Django v1.0 documentation
・【Django】ユニットテストでテスト用URLパターンを追加する方法
・Python3.6を境にjson.loads()の動きが変わっていたことで1週間ハマりました ToT
テスト対象のファイル
今回用意したテスト対象のファイルは下記のビューです。JSONのcontentにNoneがないかをチェックしてみます。
def send_json(request):
json_data = {
"foods": [{
"category": "fruit",
"contents": ['banana', 'apple', 'lemon'],
"price": 300,
}, {
"category": "vagetable",
"contents": ['tomato', 'cabbage', 'pumpkin'],
"price": 200,
}]
}
return JsonResponse(json_data)
テストコード
テストコードは下の通りになります。
from django.test import TestCase
from django.test import Client
import json
class TestSendJson(TestCase):
def test_response_data(self):
client = Client()
response = client.get('/jsonapp/send_json/')
data = json.loads(response.content.decode())
for foods in data.values():
for food in foods:
for content in food['contents']:
assert content is not None
ログインが必要な時は下記の通りに記載してあげる事でログイン可能です。
User.objects.create_user(username=username, password=password)
client.login(username=username, password=password)
ディスカッション
コメント一覧
まだ、コメントがありません