promise の挙動を少々まとめてみる
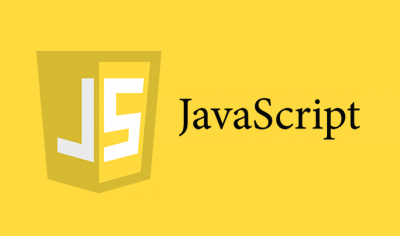
参考
非同期
下記のコードを実行すると world! のアラートが先に実行され hello のアラートが後から実行されます。
<script>
setTimeout(() => console.log('hello'), 3000);
console.log('world!');
</script>
promise
先に hello のアラートを処理して world! を後から処理したい時に promise を使って処理すると下記のようにかけるとの事。
<script>
const promise = new Promise((resolve, reject) => { // 1. Promise オブジェクトを生成して、
setTimeout(() => { // 2. 実行したい処理 3000 ミリ秒待ってから、
alert('hello'); // 3. 'hello' を呼び出して、
resolve(); // 4. 処理を resolve で終了させると、
}, 3000);
});
promise.then(
() => alert('world!') // 5. promise.then が呼び出される。
);
</script>
resolve
resolve を実行し then に処理を移すときに引数を渡す事もできるよう。
<script>
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
alert('hello');
resolve('world!'); // 1. 'world!' を promise.then に渡し、
}, 3000);
});
promise.then(
(value) => alert(value) // 'world' を変数 value が受け取る。
);
</script>
reject
resolve の代わりに reject を使用すると catch の方が処理されるよう。
<script>
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
alert('hello');
reject(); // 1. 処理を reject で終了させると、
}, 3000);
});
promise.then(
() => alert('world!')
).catch(
() => alert('errorWorld!') // 2. catch が呼び出される。
);
</script>
promise 自体を return する
promise 自体を return で返してあげる事で、[関数].then() と書く事もできる。
<script>
function saySomething (){
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
alert('hello');
resolve();
}, 3000);
});
return promise; // 1. promise を返して、
};
saySomething()
.then(() => alert("world") // 2. ここで promise.then を使用する。
);
</script>
一つずつ順番に処理
次のコードのように一つずつ順番に処理が出来るよう。
<script>
function saySomething(word) {
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
alert(word)
resolve();
}, 3000);
});
return promise;
}
saySomething('hello') // 1. hello を呼び出し、
.then(() => saySomething('earth')) // 2. hello が成功したら earth を呼び出す。
.then(() => saySomething('world')) // 3. earth が成功したら world を呼び出す。
.then(() => saySomething('cosmo')); // 4. world が成功したら cosmo を呼び出す。
</script>
ディスカッション
コメント一覧
まだ、コメントがありません