コマンドと python で S3 バケット操作
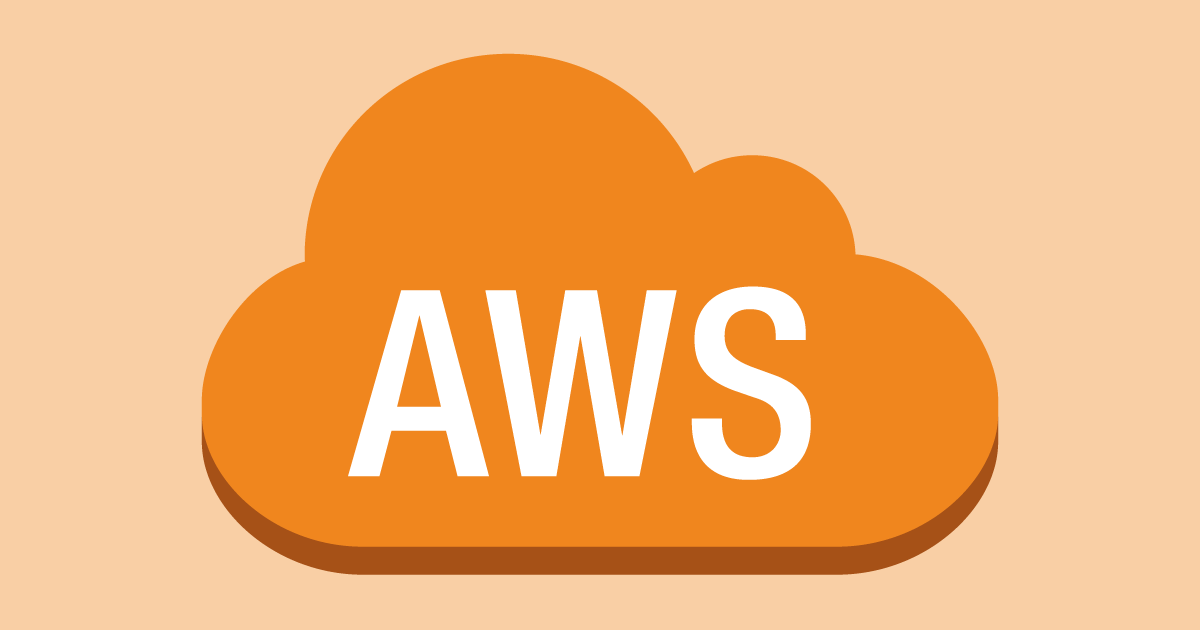
コマンドラインと python での S3 バケット操作を書き留めておこうと思います。
参考
バケットを作成する
aws s3 mb s3://hogehogebucket01
バケット一覧を表示する
aws s3 ls
ファイルをアップロードする
aws s3 cp hogehogetext.txt s3://hogehogebucket01
ファイルをダウンロードする
aws s3 cp s3://hogehogebucket01/hogehogetext.txt ./
ファイルを一覧を表示する
aws s3 ls s3://hogehogebucket01
ファイルを削除する
aws s3 rm s3://hogehogebucket01/hogehogetext.txt
バケットを削除する
# オプション --force は中身があっても強制で削除する。
aws s3 rb s3://hogehogebucket01 --force
boto3 をインストールする
pip install boto3
boto3 でバケット一覧を表示する
import boto3
s3 = boto3.resource('s3')
for bucket in s3.buckets.all():
print(bucket.name)
boto3 でファイルを一覧を表示する
import boto3
bucket_name = "hogehogebucket01"
s3 = boto3.resource('s3')
my_bucket = s3.Bucket(bucket_name)
for object in my_bucket.objects.all():
print(object)
print(object.key)
boto3 でファイルをアップロードする
import boto3
bucket_name = "hogehogebucket01"
s3 = boto3.resource('s3')
s3.Bucket(bucket_name).upload_file('hogehogetext.txt', 'hogehogetext.txt')
boto3 でファイルを削除する
import boto3
bucket_name = "hogehogebucket01"
s3_client = boto3.client('s3')
s3_client.delete_object(Bucket=bucket_name, Key='hogehogetext.txt')
boto3 でファイルをダウンロードする
import boto3
bucket_name = "hogehogebucket01"
s3 = boto3.resource('s3')
s3.Bucket(bucket_name).download_file(
'hogehogetext.txt', 'hogehogetext.txt')
boto3 で json を作成する
import boto3
import json
bucket_name = "hogehogebucket01"
file_name = "hogehoge.json"
s3 = boto3.resource('s3')
obj = s3.Object(bucket_name, file_name)
test_json = {
'key1': 'fugafuga',
'key2': 'piyopiyo'
}
data = json.dumps(test_json).encode('utf-8')
result = obj.put(Body=data)
# 書き込んだ中身を表示する。
print(obj.get()['Body'].read())
ディスカッション
コメント一覧
まだ、コメントがありません