django で CSV をダウンロードと zip してダウンロードのメモ
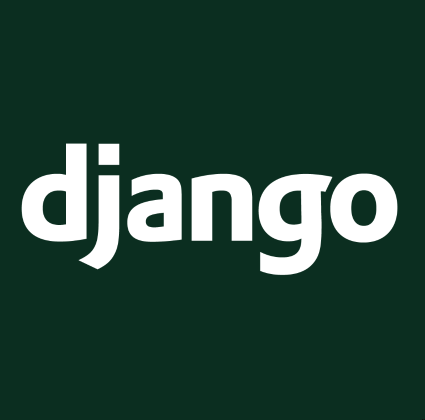
参考
インポートする
import io
import csv
import zipfile
from urllib.parse import quote
CSV をダウンロードする
def export_csv(request):
rows = [
{'column1': 1, 'column2': 2, 'column3': 3},
{'column1': 4, 'column2': 5, 'column3': 6},
{'column1': 7, 'column2': 8, 'column3': 9},
]
filename = "hogehoge.csv"
response = HttpResponse(content_type='text/csv')
response['Content-Disposition'] = "attachment;filename*=utf-8''{}".format(
quote(filename, safe=''))
writer = csv.writer(response)
writer.writerow(rows[0].keys())
[writer.writerow(row.values()) for row in rows]
return response
CSV を zip してダウンロードする
def export_zip_csv(request):
rows = [
{'column1': 1, 'column2': 2, 'column3': 3},
{'column1': 4, 'column2': 5, 'column3': 6},
{'column1': 7, 'column2': 8, 'column3': 9},
]
zip_filename = 'hogehoge.zip'
response = HttpResponse(content_type='application/zip')
response['Content-Disposition'] = "attachment;filename*=utf-8''{}".format(
quote(zip_filename, safe=''))
z = zipfile.ZipFile(response, 'w')
output1 = io.StringIO()
writer1 = csv.writer(output1, dialect='excel')
writer1.writerow(rows[0].keys())
[writer1.writerow(row.values()) for row in rows]
z.writestr("egg.csv", output1.getvalue())
output2 = io.StringIO()
writer2 = csv.writer(output2, dialect='excel')
writer2.writerow(rows[0].keys())
[writer2.writerow(row.values()) for row in rows]
z.writestr("ham.csv", output2.getvalue())
output3 = io.StringIO()
writer3 = csv.writer(output3, dialect='excel')
writer3.writerow(rows[0].keys())
[writer3.writerow(row.values()) for row in rows]
z.writestr("spam.csv", output3.getvalue())
return response
ループさせると次のとおり。
def export_zip_csv(request):
data = [
[
{'column1': 1, 'column2': 2, 'column3': 3},
{'column1': 4, 'column2': 5, 'column3': 6},
{'column1': 7, 'column2': 8, 'column3': 9},
],
[
{'column1': 11, 'column2': 22, 'column3': 33},
{'column1': 44, 'column2': 55, 'column3': 66},
{'column1': 77, 'column2': 88, 'column3': 99},
],
[
{'column1': 111, 'column2': 222, 'column3': 333},
{'column1': 444, 'column2': 555, 'column3': 666},
{'column1': 777, 'column2': 888, 'column3': 999},
],
]
zip_filename = 'hogehoge.zip'
response = HttpResponse(content_type='application/zip')
response['Content-Disposition'] = "attachment;filename*=utf-8''{}".format(
quote(zip_filename, safe=''))
z = zipfile.ZipFile(response, 'w')
csv_filenames = ['egg.csv', 'ham.csv', 'spam.csv']
for csv_filename, rows in zip(csv_filenames, data):
output = io.StringIO()
writer = csv.writer(output, dialect='excel')
writer.writerow(rows[0].keys())
[writer.writerow(row.values()) for row in rows]
z.writestr(csv_filename, output.getvalue())
return response
ディスカッション
コメント一覧
まだ、コメントがありません