python でプロセス名を変更してみる
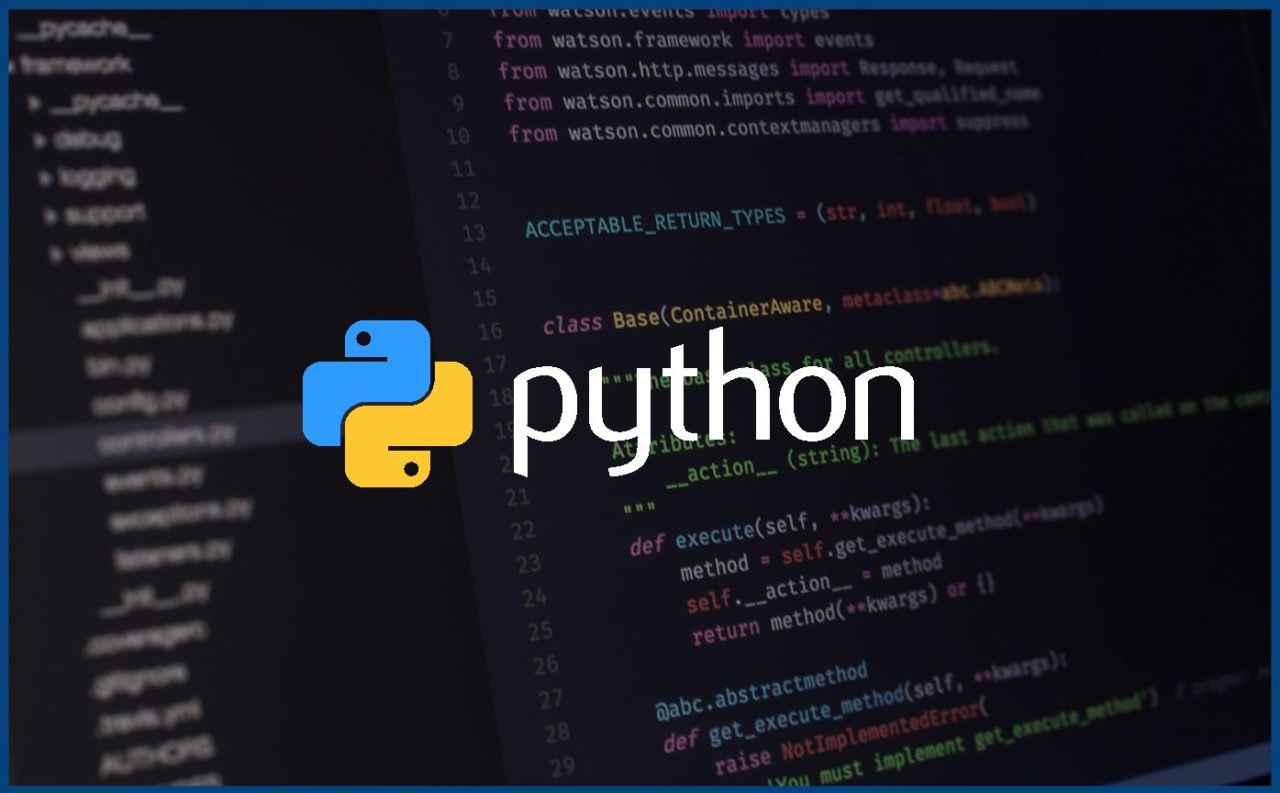
python でプロセス名を変更してみる
先日 concurrent.futures を使って並列処理をしてみました。
その時に setproctitle と言うプロセス名を変更出来るライブラリを見つけたので使ってみたいと思います。
またせっかくなので python で起動しているプロセス名を取得してみます。
参考
インストール
プロセス名を取得と変更が出来るライブラリをインストールする。
pip install setproctitle
コード
並列処理実行し新たにプロセスを起動するプログラムを用意する。
ここでは run.py としてみます。
import os
import time
from concurrent.futures import ThreadPoolExecutor, ProcessPoolExecutor
from setproctitle import setproctitle, getproctitle
def func_1(alphabet):
setproctitle(f'PROCESS_{alphabet}')
print(f'START_{getproctitle()}')
for _ in range(10):
time.sleep(1)
def main():
with ProcessPoolExecutor() as executor:
executor.map(func_1, ['A', 'B', 'C', 'D'])
if __name__ == '__main__':
main()
起動しているプロセス名を取得するプログラムを用意する。
ここでは check.py としてみます。
import psutil
def main():
running_process_names = [proc.info['name'] for proc in psutil.process_iter(
attrs=('name', 'pid'))]
print(running_process_names)
if __name__ == '__main__':
main()
試してみる
まずは、check.py だけ実行した時の結果。
['sh', 'uwsgi', 'uwsgi', 'bash', 'bash', 'bash', 'python']
run.py が動いている間に check.py を実行した結果。
['sh', 'uwsgi', 'uwsgi', 'bash', 'bash', 'bash', 'python', 'PROCESS_A', 'PROCESS_B', 'PROCESS_C', 'PROCESS_D', 'python', 'python', 'python', 'python', 'python']
run.py が動いている間に ps aux | grep PROCESS を事項した結果。
root 591 0.0 0.1 14176 9416 pts/2 S+ 13:47 0:00 PROCESS_A
root 592 0.0 0.1 14176 9416 pts/2 S+ 13:47 0:00 PROCESS_B
root 593 0.0 0.1 14176 9416 pts/2 S+ 13:47 0:00 PROCESS_C
root 594 0.0 0.1 14432 9424 pts/2 S+ 13:47 0:00 PROCESS_D
root 602 0.0 0.0 5064 716 pts/3 S+ 13:47 0:00 grep PROCESS
ディスカッション
コメント一覧
まだ、コメントがありません